<< RETURN: Coding / Kotlin / Coding with Kotlin
Introduction
Compose Multiplatform by Jetbrains is basically Jetpack Compose for multiple platforms (Desktop, iOS, Web). It is a declarative framework which accelerates application UI development.
Getting Started
To start you’ll need an IDE for creating a Compose Multiplatform project. I’ll be using IntelliJ IDEA but feel free to use whichever works for you. If you’re not familiar with IntelliJ IDEA, you can go to Getting Started to familiarize yourself with coding using IntelliJ IDEA.
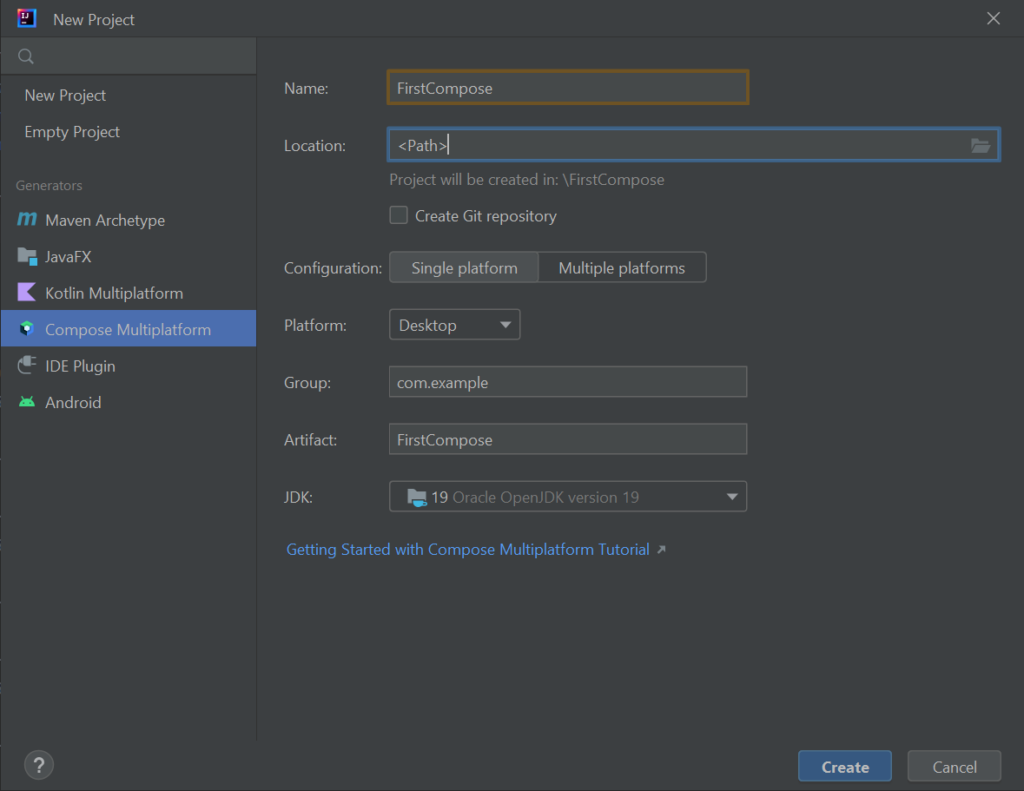
On IntelliJ, select new project and the above dialog prompt should appear. At this point, you need to select Compose Multiplatform and if you’re going for a Single platform configuration, be sure to select the Platform (Desktop/Web).
Example
@Composable
@Preview
fun app() {
var text by remember { mutableStateOf("Hello, World!") }
MaterialTheme {
Column(
modifier = Modifier.fillMaxSize().padding(0.dp, 10.dp),
horizontalAlignment = Alignment.CenterHorizontally) {
TextField(
value = text,
onValueChange = { text = it },
label = { Text("My Label") },
textStyle = TextStyle(color = Color.Red, fontFamily = FontFamily.Cursive),
readOnly = true
)
Button(onClick = {
text = "Hello, Desktop!"
}) {
Text(text)
}
Button(onClick = {
text = "Hello, World!"
}) {
Text("Reset")
}
Button(onClick = {
text = "${1+1}"
}) {
Text("1 + 1")
}
}
}
}
fun main() = application {
Window(onCloseRequest = ::exitApplication, title = "Compose for Desktop") {
app()
}
}
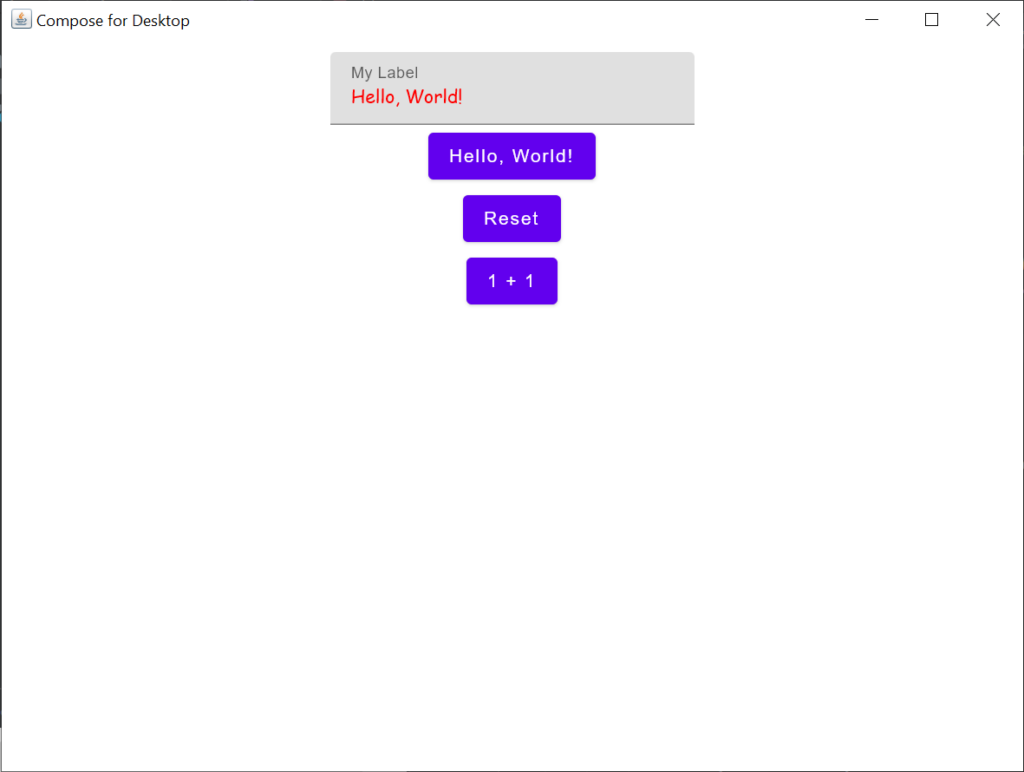
For a more detailed step by step guide, you can go to Jetbrains Github repository.
Desktop Preview
In IntelliJ, you can view how the UI looks as you code. All you need is to install the Plugin Compose Multiplatform IDE Support. This can be done by going to Settings (Ctrl + Alt + S), go to Plugins, and install Compose Multiplatform IDE Support.
After installing the plugin, there should be a Jetpack Compose icon to the left of the function which has a @Preview
notation written. If you click on it, a desktop preview will appear and you can dock/pin it to your IDE. Anytime there is a change in the UI, clicking on this icon will refresh your preview.
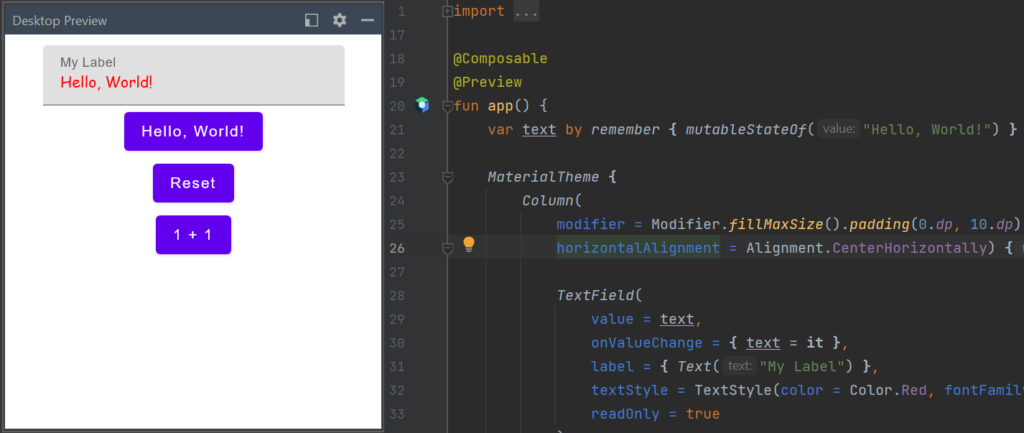
Next Up
Once you’re done with Compose Multiplatform, you can see how to build a Simple Calculator using Compose Multiplatform.